To replace words in a paragraph using JavaScript, you can follow these steps:
- Start by obtaining the paragraph element from the HTML document. This can be done using various methods, such as querying the element by its ID, class, or tag name.
- Use the innerHTML property of the paragraph element to access and modify its content. This property allows you to retrieve or update the HTML content within the element. In this case, you will use it to replace certain words.
- Define the words you want to replace and their corresponding replacements. You can store them in variables or directly write them as strings.
- Utilize the replace() method available for string objects in JavaScript. This method allows you to replace occurrences of a specified string with another string.
- Apply the replace() method to the paragraph's innerHTML, specifying the word to be replaced as the first argument and its replacement as the second argument. Save the modified content back into the innerHTML to update the paragraph.
Here's an example code snippet demonstrating the process:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
// Step 1: Obtain the paragraph element var paragraph = document.getElementById("myParagraph"); // Step 2: Access and modify the paragraph's content var content = paragraph.innerHTML; // Step 3: Define words and replacements var wordToReplace = "old"; var replacementWord = "new"; // Step 4: Use replace() method to replace words var modifiedContent = content.replace(wordToReplace, replacementWord); // Step 5: Update the paragraph with modified content paragraph.innerHTML = modifiedContent; |
In this example, we replace the word "old" with "new" in the paragraph element with the ID "myParagraph". You can adjust the code according to your specific needs, such as replacing multiple words or handling case sensitivity.
Best JavaScript Books to Read in 2024
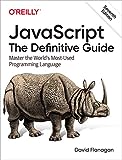
Rating is 5 out of 5
JavaScript: The Definitive Guide: Master the World's Most-Used Programming Language
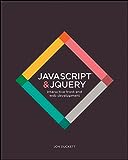
Rating is 4.8 out of 5
JavaScript and jQuery: Interactive Front-End Web Development
- JavaScript Jquery
- Introduces core programming concepts in JavaScript and jQuery
- Uses clear descriptions, inspiring examples, and easy-to-follow diagrams
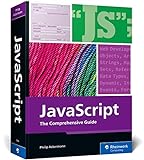
Rating is 4.7 out of 5
JavaScript: The Comprehensive Guide to Learning Professional JavaScript Programming (The Rheinwerk Computing)
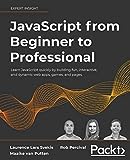
Rating is 4.6 out of 5
JavaScript from Beginner to Professional: Learn JavaScript quickly by building fun, interactive, and dynamic web apps, games, and pages
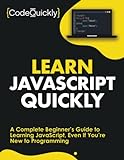
Rating is 4.4 out of 5
Learn JavaScript Quickly: A Complete Beginner’s Guide to Learning JavaScript, Even If You’re New to Programming (Crash Course With Hands-On Project)
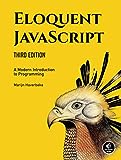
Rating is 4.3 out of 5
Eloquent JavaScript, 3rd Edition: A Modern Introduction to Programming
- It can be a gift option
- Comes with secure packaging
- It is made up of premium quality material.
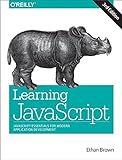
Rating is 4.1 out of 5
Learning JavaScript: JavaScript Essentials for Modern Application Development
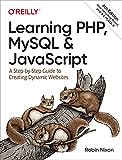
Rating is 4 out of 5
Learning PHP, MySQL & JavaScript: A Step-by-Step Guide to Creating Dynamic Websites (Learning PHP, MYSQL, Javascript, CSS & HTML5)
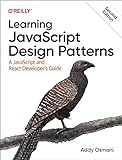
Rating is 3.9 out of 5
Learning JavaScript Design Patterns: A JavaScript and React Developer's Guide
Can you replace words in a paragraph based on their position within the paragraph?
Yes, I can replace words in a paragraph based on their position within the paragraph. Please provide me with the paragraph and let me know which specific words you would like to replace, along with the replacements you have in mind.
How can you replace a specific word in a paragraph using JavaScript?
To replace a specific word in a paragraph using JavaScript, you can make use of the String's replace()
method. The replace()
method can replace a specified value with another value in a string.
Here is an example of how you can replace a word in a paragraph:
1 2 3 4 5 6 |
let paragraph = "I like apples. Apples are tasty."; // Replace "apples" with "oranges" in the paragraph let replacedParagraph = paragraph.replace("apples", "oranges"); console.log(replacedParagraph); |
In this example, the replace()
method is called on the paragraph
string. It replaces all occurrences of the word "apples" with the word "oranges". The updated paragraph is stored in the replacedParagraph
variable.
The output of the above code will be:
1
|
I like oranges. Oranges are tasty.
|
You can modify the code according to your requirements by changing the words you want to replace.
Can you replace words in a paragraph based on their semantic meaning rather than exact matches?
Yes, it is possible to replace words in a paragraph based on their semantic meaning rather than exact matches. This process is commonly known as paraphrasing. By understanding the context and the meaning conveyed by particular words or phrases, one can find alternative expressions that convey the same message using different words. However, it is important to note that paraphrasing should be done carefully to maintain the accuracy and intent of the original text.