To sort tooltip values in Chart.js, you can use the tooltips.callbacks.label
function to customize the tooltip label. Within this function, you can access the tooltip item array and sort the values as needed before displaying them in the tooltip. By sorting the tooltip values, you can present the data in a more organized and meaningful way for the users.
Best JavaScript Books to Read in 2024
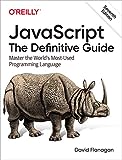
Rating is 5 out of 5
JavaScript: The Definitive Guide: Master the World's Most-Used Programming Language
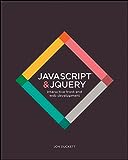
Rating is 4.8 out of 5
JavaScript and jQuery: Interactive Front-End Web Development
- JavaScript Jquery
- Introduces core programming concepts in JavaScript and jQuery
- Uses clear descriptions, inspiring examples, and easy-to-follow diagrams
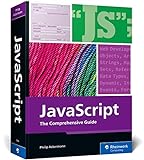
Rating is 4.7 out of 5
JavaScript: The Comprehensive Guide to Learning Professional JavaScript Programming (The Rheinwerk Computing)
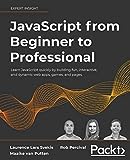
Rating is 4.6 out of 5
JavaScript from Beginner to Professional: Learn JavaScript quickly by building fun, interactive, and dynamic web apps, games, and pages
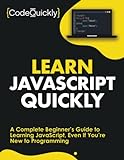
Rating is 4.4 out of 5
Learn JavaScript Quickly: A Complete Beginner’s Guide to Learning JavaScript, Even If You’re New to Programming (Crash Course With Hands-On Project)
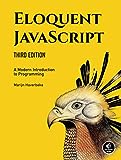
Rating is 4.3 out of 5
Eloquent JavaScript, 3rd Edition: A Modern Introduction to Programming
- It can be a gift option
- Comes with secure packaging
- It is made up of premium quality material.
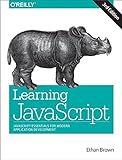
Rating is 4.1 out of 5
Learning JavaScript: JavaScript Essentials for Modern Application Development
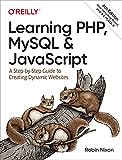
Rating is 4 out of 5
Learning PHP, MySQL & JavaScript: A Step-by-Step Guide to Creating Dynamic Websites (Learning PHP, MYSQL, Javascript, CSS & HTML5)
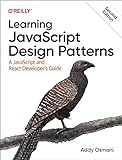
Rating is 3.9 out of 5
Learning JavaScript Design Patterns: A JavaScript and React Developer's Guide
How to customize the sorting function for tooltip values in chart.js?
To customize the sorting function for tooltip values in Chart.js, you can use the tooltips.callbacks.label
function. This function allows you to define custom sorting logic for the tooltip labels.
Here is an example of how you can customize the sorting function for tooltip values in Chart.js:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
var myChart = new Chart(ctx, { type: 'bar', data: { labels: ["A", "B", "C", "D", "E"], datasets: [{ label: 'Data', data: [10, 20, 15, 25, 30], backgroundColor: 'rgba(255, 99, 132, 0.2)', borderColor: 'rgba(255, 99, 132, 1)', borderWidth: 1 }] }, options: { tooltips: { callbacks: { label: function(tooltipItem, data) { var dataset = data.datasets[tooltipItem.datasetIndex]; var value = dataset.data[tooltipItem.index]; var sortedData = dataset.data.slice().sort((a, b) => a - b); var label = data.labels[tooltipItem.index] + ': ' + value; // Custom sorting logic var sortedIndex = sortedData.indexOf(value); return label + ' (Sorted Index: ' + sortedIndex + ')'; } } } } }); |
In this example, we have defined a custom sorting logic inside the tooltips.callbacks.label
function. We first retrieve the value of the tooltip item and create a sorted copy of the dataset data array. We then find the index of the value in the sorted array and append it to the tooltip label. This way, we can customize the sorting function for the tooltip values in Chart.js.
What are the different options for sorting tooltip values in chart.js?
There are various options for sorting tooltip values in chart.js, including:
- Ascending: Display tooltip values in ascending order.
- Descending: Display tooltip values in descending order.
- Nearest: Display the tooltip value closest to the mouse cursor.
- Range: Display tooltip values within a specified range.
- Custom: Allows for custom sorting of tooltip values based on user-defined criteria.
These options can be set using the "mode" property in the tooltip configuration section of the chart.js settings.
What is the default sorting method for tooltip values in chart.js?
The default sorting method for tooltip values in chart.js is to sort them in ascending order.
How to reset the sorting of tooltip values in chart.js?
To reset the sorting of tooltip values in a chart created with Chart.js, you can use the following steps:
- Get the reference to the chart instance that you want to reset the tooltip sorting for.
1 2 3 4 5 6 7 |
var myChart = new Chart(ctx, { type: 'bar', data: data, options: options }); var chartInstance = myChart; |
- Access the options object of the chart instance and set the tooltip mode to 'index'.
1
|
chartInstance.options.tooltips.mode = 'index';
|
- Update the chart instance to apply the changes.
1
|
chartInstance.update();
|
By setting the tooltip mode to 'index', the tooltip values will be displayed in the same order as the data points in the dataset, effectively resetting the sorting.