In JavaScript, you can convert a number to a string using the toString()
method or by concatenating an empty string with the number.
The toString()
method converts a number to its equivalent string representation. For example, if you have a number num
and you want to convert it to a string, you can use num.toString()
. This will return a string representation of the number.
Another way to convert a number to a string is by concatenating it with an empty string. This coerces the number into a string. For example, if you have a number num
and you want to convert it to a string, you can use "" + num
. The empty string serves as a placeholder, and when it is added to the number, JavaScript automatically converts the number to a string.
Here's an example:
1 2 3 4 5 6 |
let num = 42; let str = num.toString(); console.log(str); // Output: "42" let str2 = "" + num; console.log(str2); // Output: "42" |
Both methods will successfully convert a number to a string in JavaScript. Choose the method that suits your needs and coding style.
Best JavaScript Books to Read in 2025
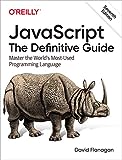
Rating is 5 out of 5
JavaScript: The Definitive Guide: Master the World's Most-Used Programming Language
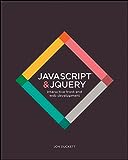
Rating is 4.8 out of 5
JavaScript and jQuery: Interactive Front-End Web Development
- JavaScript Jquery
- Introduces core programming concepts in JavaScript and jQuery
- Uses clear descriptions, inspiring examples, and easy-to-follow diagrams
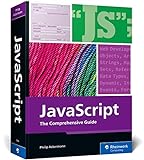
Rating is 4.7 out of 5
JavaScript: The Comprehensive Guide to Learning Professional JavaScript Programming (The Rheinwerk Computing)
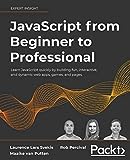
Rating is 4.6 out of 5
JavaScript from Beginner to Professional: Learn JavaScript quickly by building fun, interactive, and dynamic web apps, games, and pages
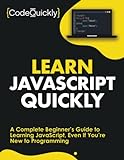
Rating is 4.4 out of 5
Learn JavaScript Quickly: A Complete Beginner’s Guide to Learning JavaScript, Even If You’re New to Programming (Crash Course With Hands-On Project)
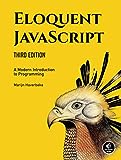
Rating is 4.3 out of 5
Eloquent JavaScript, 3rd Edition: A Modern Introduction to Programming
- It can be a gift option
- Comes with secure packaging
- It is made up of premium quality material.
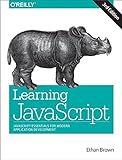
Rating is 4.1 out of 5
Learning JavaScript: JavaScript Essentials for Modern Application Development
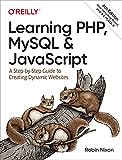
Rating is 4 out of 5
Learning PHP, MySQL & JavaScript: A Step-by-Step Guide to Creating Dynamic Websites (Learning PHP, MYSQL, Javascript, CSS & HTML5)
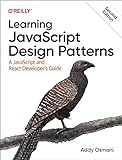
Rating is 3.9 out of 5
Learning JavaScript Design Patterns: A JavaScript and React Developer's Guide
Can you provide an example of converting a float to a string with a fixed number of decimal places?
Sure! Here's an example in Python:
1 2 3 4 5 6 |
number = 4.56789 decimal_places = 2 string_number = "{:.{}f}".format(number, decimal_places) print(string_number) |
Output:
1
|
4.57
|
Explanation:
In this example, we have a float number 4.56789
and we want to convert it to a string representation with 2 decimal places. The {:.{}f}
format specifier is used to achieve this. The :.{}f
part specifies the format for the floating-point number, where the second placeholder {}
is replaced with the value of decimal_places
. The format()
function is used to format the number and convert it to a string. Finally, the string_number
variable contains the desired result.
Can you convert a negative number to a string while preserving the negative sign in JavaScript?
Yes, you can convert a negative number to a string while preserving the negative sign in JavaScript. One way to do this is by using the toString()
method and concatenating the negative sign manually. Here's an example:
1 2 3 4 |
let number = -42; let string = "-" + Math.abs(number).toString(); console.log(string); // Output: "-42" |
In this example, Math.abs()
is used to get the absolute value of the negative number, which removes the negative sign. Then, the negative sign is concatenated with the resulting absolute value to preserve the negative sign when converting it to a string.
What happens if you try to convert a NaN (Not a Number) value to a string in JavaScript?
If you try to convert a NaN (Not a Number) value to a string in JavaScript using the toString()
method, it will return the string representation of the NaN value. The result will be the string "NaN".