Reading a JSON file in JavaScript involves a few key steps. Here's how it can be done:
- Fetch the JSON file: Use the fetch() function to retrieve the JSON file from a server or local file system. This function returns a Promise that resolves to the Response object.
1 2 3 4 |
fetch('file.json') .then(function(response) { // Code to handle the response }); |
- Parse the JSON: Extract the JSON data from the Response object and use the json() method to parse it into a JavaScript object or array.
1 2 3 4 5 6 7 |
fetch('file.json') .then(function(response) { return response.json(); // Parse the JSON data }) .then(function(data) { // Code to handle the parsed JSON data }); |
- Access the data: Once parsed, you can access the data within the JavaScript object or array as desired.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
fetch('file.json') .then(function(response) { return response.json(); }) .then(function(data) { // Access data properties console.log(data.property1); console.log(data.property2); // Access array elements console.log(data.array[0]); console.log(data.array[1]); }); |
Note that file.json
should be replaced with the actual path or URL of the JSON file you want to read. Additionally, appropriate error handling should be implemented to handle any unsuccessful responses or parsing errors that may occur.
Best JavaScript Books to Read in 2025
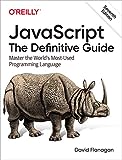
Rating is 5 out of 5
JavaScript: The Definitive Guide: Master the World's Most-Used Programming Language
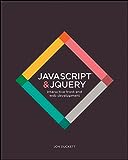
Rating is 4.8 out of 5
JavaScript and jQuery: Interactive Front-End Web Development
- JavaScript Jquery
- Introduces core programming concepts in JavaScript and jQuery
- Uses clear descriptions, inspiring examples, and easy-to-follow diagrams
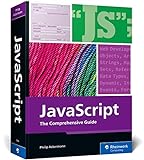
Rating is 4.7 out of 5
JavaScript: The Comprehensive Guide to Learning Professional JavaScript Programming (The Rheinwerk Computing)
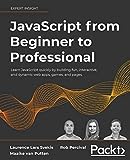
Rating is 4.6 out of 5
JavaScript from Beginner to Professional: Learn JavaScript quickly by building fun, interactive, and dynamic web apps, games, and pages
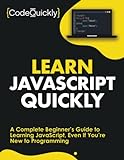
Rating is 4.4 out of 5
Learn JavaScript Quickly: A Complete Beginner’s Guide to Learning JavaScript, Even If You’re New to Programming (Crash Course With Hands-On Project)
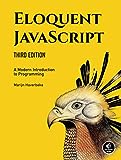
Rating is 4.3 out of 5
Eloquent JavaScript, 3rd Edition: A Modern Introduction to Programming
- It can be a gift option
- Comes with secure packaging
- It is made up of premium quality material.
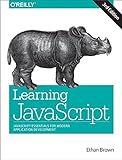
Rating is 4.1 out of 5
Learning JavaScript: JavaScript Essentials for Modern Application Development
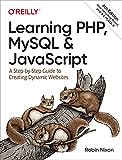
Rating is 4 out of 5
Learning PHP, MySQL & JavaScript: A Step-by-Step Guide to Creating Dynamic Websites (Learning PHP, MYSQL, Javascript, CSS & HTML5)
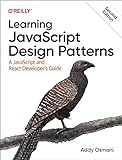
Rating is 3.9 out of 5
Learning JavaScript Design Patterns: A JavaScript and React Developer's Guide
How do you read a JSON file synchronously in JavaScript?
In JavaScript, you can read a JSON file synchronously using the fs
module provided by Node.js. Here's an example of how you can achieve it:
- First, make sure you have the fs module installed by requiring it at the top of your script:
1
|
const fs = require('fs');
|
- Use the fs.readFileSync() method to read the JSON file synchronously. This method takes two parameters: the path to the JSON file and the character encoding (usually 'utf8').
1
|
const rawData = fs.readFileSync('path/to/file.json', 'utf8');
|
- Parse the raw data using JSON.parse() to convert it into a JavaScript object:
1
|
const jsonData = JSON.parse(rawData);
|
Now, you can use the jsonData
object to access the values in the JSON file.
It's important to note that synchronous file reading can block the execution of other code in the event loop, so it's usually recommended to use asynchronous methods (fs.readFile()
) instead to avoid blocking.
Can you read a JSON file using jQuery?
Yes, you can read a JSON file using jQuery. Here's an example of how you can do it:
1 2 3 4 |
$.getJSON('file.json', function(data) { // 'data' will contain the JSON data from the file console.log(data); }); |
In the example above, you use the $.getJSON()
function in jQuery to make a GET request to the JSON file. The callback function will be executed when the request is successful, and the JSON data will be passed as the data
parameter.
Can you read nested JSON objects in JavaScript?
Yes, it is possible to read nested JSON objects in JavaScript.
To access nested values in a JSON object, you can use the dot notation (.) or bracket notation ([]). Here's an example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
var data = { "name": "John", "age": 25, "address": { "street": "123 Main St", "city": "Exampleville", "country": "USA" } }; // Accessing nested values using dot notation console.log(data.name); // Output: John console.log(data.address.city); // Output: Exampleville // Accessing nested values using bracket notation console.log(data['name']); // Output: John console.log(data['address']['city']); // Output: Exampleville |
You can access nested JSON objects by chaining the dot or bracket notation according to the structure of the JSON object.