In JavaScript, you can use the window.location
property to redirect to another page by assigning a new URL to it. Here is the syntax you can use:
1
|
window.location.href = "newPage.html";
|
This line of code will redirect the user to the specified URL, in this case, "newPage.html". You can replace "newPage.html" with any other valid URL.
You can also use the window.location.replace()
method to achieve the same result. Here is an example:
1
|
window.location.replace("newPage.html");
|
Both methods perform a redirect, but the difference is that the replace()
method removes the current URL from the browser history, while the href
property adds a new entry to the history.
It's important to note that JavaScript page redirects should generally be used sparingly and with caution, as excessive or unnecessary redirects can cause a poor user experience.
Best JavaScript Books to Read in 2024
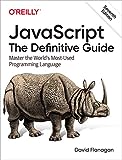
Rating is 5 out of 5
JavaScript: The Definitive Guide: Master the World's Most-Used Programming Language
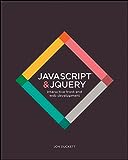
Rating is 4.8 out of 5
JavaScript and jQuery: Interactive Front-End Web Development
- JavaScript Jquery
- Introduces core programming concepts in JavaScript and jQuery
- Uses clear descriptions, inspiring examples, and easy-to-follow diagrams
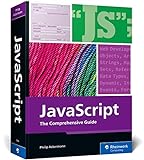
Rating is 4.7 out of 5
JavaScript: The Comprehensive Guide to Learning Professional JavaScript Programming (The Rheinwerk Computing)
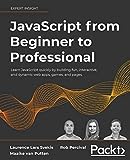
Rating is 4.6 out of 5
JavaScript from Beginner to Professional: Learn JavaScript quickly by building fun, interactive, and dynamic web apps, games, and pages
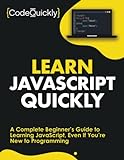
Rating is 4.4 out of 5
Learn JavaScript Quickly: A Complete Beginner’s Guide to Learning JavaScript, Even If You’re New to Programming (Crash Course With Hands-On Project)
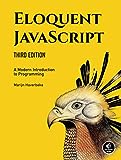
Rating is 4.3 out of 5
Eloquent JavaScript, 3rd Edition: A Modern Introduction to Programming
- It can be a gift option
- Comes with secure packaging
- It is made up of premium quality material.
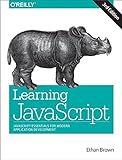
Rating is 4.1 out of 5
Learning JavaScript: JavaScript Essentials for Modern Application Development
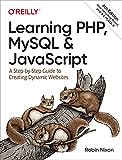
Rating is 4 out of 5
Learning PHP, MySQL & JavaScript: A Step-by-Step Guide to Creating Dynamic Websites (Learning PHP, MYSQL, Javascript, CSS & HTML5)
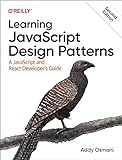
Rating is 3.9 out of 5
Learning JavaScript Design Patterns: A JavaScript and React Developer's Guide
How do you redirect to a different page after a form submission?
To redirect to a different page after a form submission, you can use one of the following methods:
- Using the HTML form tag: In this method, the action attribute of the form tag specifies the URL of the page where the form data should be submitted. Once the form is submitted, the browser will automatically navigate to the specified page.
- Using JavaScript: In this method, the onsubmit event of the form tag is intercepted using a JavaScript function. The event.preventDefault() method prevents the default form submission behavior. After handling the form submission, you can use window.location.href to redirect the user to the desired page.
- Using server-side programming: If you are using server-side programming, such as PHP, you can handle the form submission on the server and then use server-side code to redirect to a different page. For example, in PHP: After processing the form data, you can use header("Location: your_target_page.php") to redirect the user to the desired page. The exit function ensures that the script execution ends immediately after the redirect.
Choose the method that suits your needs and implement it based on your programming environment.
What is JavaScript?
JavaScript is a high-level programming language that is primarily used to create interactive web pages and web applications. It is often abbreviated as JS. It allows developers to add dynamic and interactive elements to websites, such as user input forms, animations, and content updates. JavaScript can be used both on the client-side, meaning it runs on the user's web browser, and on the server-side, meaning it runs on the web server. It is one of the core technologies for web development along with HTML and CSS.
What is setTimeout() used for in JavaScript?
setTimeout() is used in JavaScript to delay the execution of a code or function for a specified amount of time. It takes two parameters: a callback function or a code to be executed, and a delay time in milliseconds. The code or function specified will be executed once after the specified delay time has passed. It is commonly used to create a delay before performing certain actions, implementing animations, or scheduling tasks that require a delay.